Authorization Rules (V1)
Learn how to create, update and apply simple rules on card transactions.
Authorization Rules (V1) has been discontinued.
Auth Rules (V1) has been superseded by a newer, improved version and V1 endpoints have been removed. The methods described on this page are no longer available. Please see Auth Rules (V2) for current information about improved Lithic-hosted Authorization Rules.
An authorization rule (Auth Rule) is a control you can set to better manage the transactions on your cards. It enables you to allow or block transactions in specific countries, and allow or block transactions at specific merchant types.
To minimize conflict, any given entity (program, account, card) that makes up your program can only have one Auth Rule directly applied to it. However, any higher-level entity's Auth Rule will apply to all entities below it - e.g., a given card's transactions will be subject to the Auth Rule applied to the account that the card belongs to AND the Auth Rule applied to the program that the card belongs to.
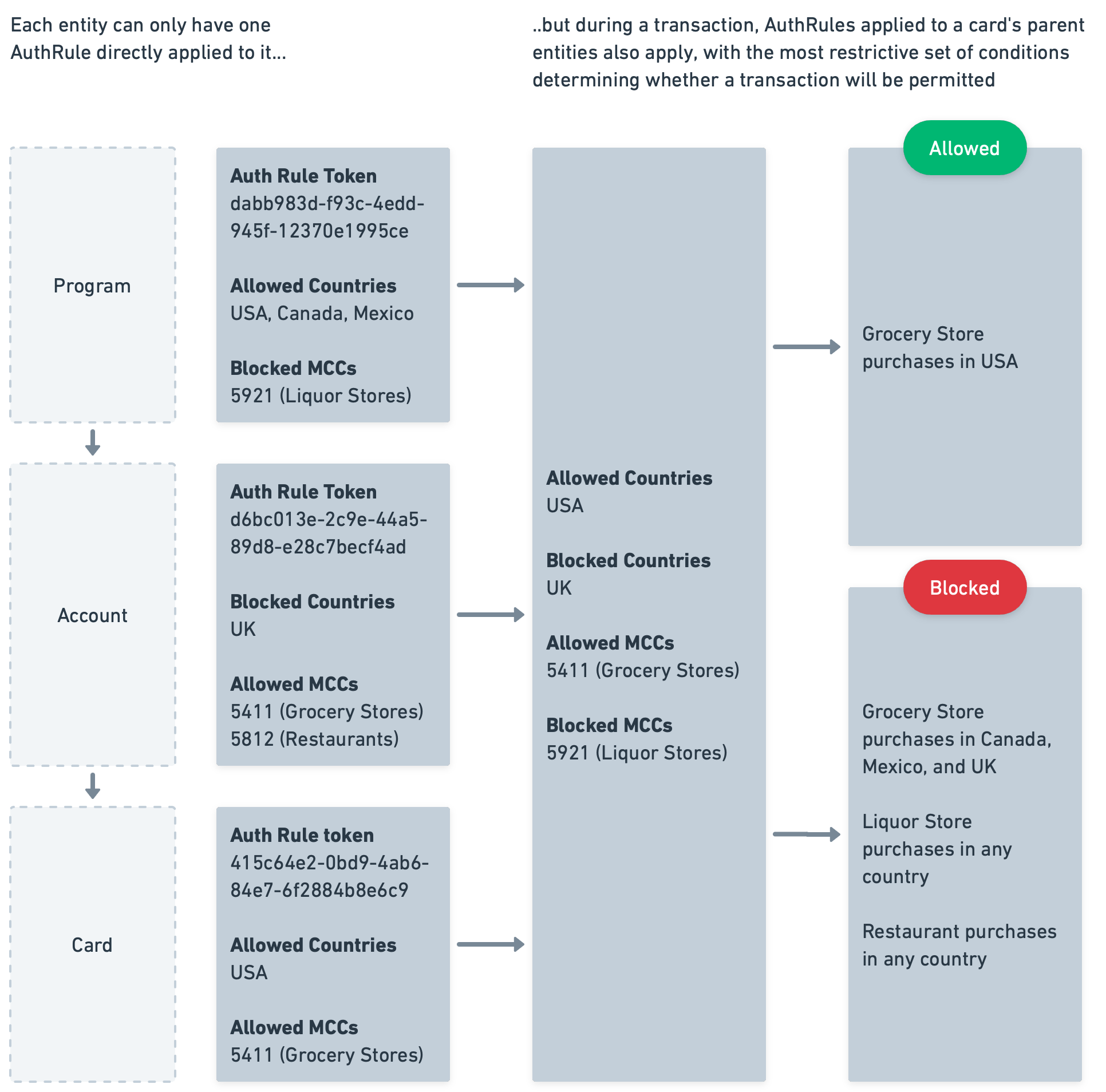
See Merchant Category Codes (MCCs) for a detailed list of MCCs that can be used in creating and modifying Auth Rules.
Auth Rule Schema
{
"token": String,
"allowed_countries": List,
"blocked_countries": List,
"allowed_mcc": List,
"blocked_mcc": List,
"state": String,
"program_level": Boolean,
"card_tokens": List,
"account_tokens": List
}
token | Globally unique identifier for the Auth Rule |
allowed_countries | Countries in which the Auth Rule will permit transactions, listed in uppercase ISO 3166-1 alpha-3 three character abbreviations. Note that Lithic maintains a list of countries in which all transactions are blocked; "allowing" those countries in an Auth Rule will not override the Lithic-wide restrictions |
blocked_countries | Countries in which the Auth Rule will decline transactions, listed in uppercase ISO 3166-1 alpha-3 three character abbreviations |
allowed_mcc | Merchant category codes for which the Auth Rule will permit transactions, listed in ISO 18245 four-digits. Note that Lithic maintains a list of MCCs for which all transactions are blocked; "allowing" those MCCs in an Auth Rule will not override the Lithic-wide restrictions |
blocked_mcc | Merchant category codes for which the Auth Rule will decline transactions, listed in ISO 18245 four-digits |
state | Indicates whether the Auth Rule is ACTIVE or INACTIVE |
program_level | Boolean indicating whether the Auth Rule will be applied at the program level |
card_tokens | Array of card_token(s) identifying the cards that the Auth Rule will apply to |
account_tokens | Array of account_token(s) identifying the accounts that the Auth Rule will apply to |
Note that the use of MCC or country restrictions beyond the terms included in your cardholder agreement with end-users may require additional steps to ensure compliance with card network policies. Use of these restrictions is an agreement from the API user that the restrictions are in compliance with your cardholder agreement terms. Reach out to your Customer Success rep for additional support.
Create Auth Rules
Creates an authorization rule and applies it at the program, account, or card level.
This endpoint will reject an attempt to create an Auth Rule that conflicts with an existing Auth Rule that applies to the target entity. For example, if a program level Auth Rule blocks transactions in Canada, and an attempt is made to create a card level Auth Rule to allow transactions in Canada (on a card belonging to that program), the POST /auth_rules call will be rejected.
POST https://api.lithic.com/v1/auth_rules
Sample Request
curl https://api.lithic.com/v1/auth_rules \
-X POST \
-H "Authorization: YOUR_API_KEY" \
-H "Content-Type: application/json" \
-d '
{
"allowed_countries":["USA"],
"allowed_mcc":["3000", "3001"],
"program_level": true
}
'
Sample Response
{
"allowed_countries": ["USA"],
"blocked_countries": [],
"allowed_mcc": ["3000", "3001"],
"blocked_mcc": [],
"token": "d39e680e-af95-47ad-a78e-2415a0c8a81f",
"program_level": true,
"state": "ACTIVE",
"card_tokens": [],
"account_tokens": []
}
To successfully create an Auth Rule, at least one type of control parameter (country control, MCC control) and only one type of entity parameter (card, account, program-level) should be included in the request.
allowed_countries (optional) | List of countries in which the Auth Rule will permit transactions. E.g., to allow transactions in the US and Canada only, input "USA" and "CAN". List. Permitted values: List of uppercase ISO 3166-1 alpha-3 three character abbreviations. Note that only this field OR blocked_countries can be used for a given Auth Rule. |
blocked_countries (optional) | List of countries in which the Auth Rule will automatically decline transactions. E.g., to block transactions in the US and Canada only, input "USA" and "CAN". List. Permitted values: List of uppercase ISO 3166-1 alpha-3 three character abbreviations. Note that only this field OR allowed_countries can be used for a given Auth Rule. |
allowed_mcc (optional) | List of merchant category codes for which the Auth Rule will permit transactions. E.g., to permit transactions only at Shoe Stores, input "5661". List. Permitted values: List of ISO 18245 four-digit MCCs. Note that only this field OR blocked_mcc can be used for a given Auth Rule. |
blocked_mcc (optional) | List of merchant category codes for which the Auth Rule will automatically decline transactions. E.g., to block transactions only at Shoe Stores, input "5661". List. Permitted values: List of ISO 18245 four-digit MCCs. Note that only this field OR allowed_mcc can be used for a given Auth Rule. |
card_tokens (optional) | List of cardtoken(s) identifying the cards that the Auth Rule will apply to. _List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, account_tokens and program_level cannot. However, one of the three parameters must be included. |
account_tokens (optional) | List of accounttoken(s) identifying the accounts that the Auth Rule will apply to. _List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, card_tokens and program_level cannot. However, one of the three parameters must be included. |
program_level (optional) | Boolean indicating whether the Auth Rule should be applied at the program level (true if yes; false if no) String. Permitted values: true , false . Note that if this parameter is included, card_tokens and account_tokens cannot. However, one of the three parameters must be included. |
Update Auth Rules
Update the properties associated with an existing authorization rule.
PUT https://api.lithic.com/v1/auth_rules/{auth_rule_token}
Sample Request
curl https://api.lithic.com/v1/auth_rules/d39e680e-af95-47ad-a78e-2415a0c8a81f \
-X PUT \
-H "Authorization: YOUR_API_KEY" \
-H "Content-Type: application/json" \
-d '
{
"allowed_countries":["USA","CAN"],
"blocked_mcc":["3000","3001"]
}
'
Sample Response
{
"allowed_countries": ["USA","CAN"],
"blocked_countries": [],
"allowed_mcc": ["3000","3001"],
"blocked_mcc": [],
"token": "d39e680e-af95-47ad-a78e-2415a0c8a81f",
"program_level": true,
"state": "ACTIVE",
"card_tokens": [],
"account_tokens": []
}
To update an Auth Rule, at least one type of control parameter (country control, MCC control) should be included in the request. Note that using this endpoint will update the entire configuration of the Auth Rule, not just the parameters passed in.
allowed_countries (optional) | List of countries in which the Auth Rule will permit transactions. E.g., to allow transactions in the US and Canada only, input "USA" and "CAN". List. Permitted values: List of uppercase ISO 3166-1 alpha-3 three character abbreviations. Note that only this field OR blocked_countries can be used for a given Auth Rule. |
blocked_countries (optional) | List of countries in which the Auth Rule will automatically decline transactions. E.g., to block transactions in the US and Canada only, input "USA" and "CAN". List. Permitted values: List of uppercase ISO 3166-1 alpha-3 three character abbreviations. Note that only this field OR allowed_countries can be used for a given Auth Rule. |
allowed_mcc (optional) | List of merchant category codes for which the Auth Rule will permit transactions. E.g., to permit transactions only at Shoe Stores, input "5661". List. Permitted values: List of ISO 18245 four-digit MCCs. Note that only this field OR blocked_mcc can be used for a given Auth Rule. |
blocked_mcc (optional) | List of merchant category codes for which the Auth Rule will automatically decline transactions. E.g., to block transactions only at Shoe Stores, input "5661". List. Permitted values: List of ISO 18245 four-digit MCCs. Note that only this field OR allowed_mcc can be used for a given Auth Rule. |
List Auth Rules
List the properties and entities (program, accounts, and cards) associated with an existing authorization rule. If no Auth Rule token is provided, this endpoint will return all of the Auth Rules under the program.
GET https://api.lithic.com/v1/auth_rules/{auth_rule_token}
Sample Request
curl https://api.lithic.com/v1/auth_rules/d39e680e-af95-47ad-a78e-2415a0c8a81f \
-H "Authorization: YOUR_API_KEY" \
-H "Content-Type: application/json"
Sample Response
{
"allowed_countries": [
"USA",
"CAN"
],
"blocked_countries": [],
"allowed_mcc": [],
"blocked_mcc": [
"3000",
"3001"
],
"token": "d39e680e-af95-47ad-a78e-2415a0c8a81f",
"program_level": true,
"state": "ACTIVE",
"card_tokens": [],
"account_tokens": []
}
Apply Auth Rule to Entity
Applies an existing authorization rule to a program, account(s), or card(s).
POST https://api.lithic.com/v1/auth_rules/{auth_rule_token}/apply
Sample Request
curl https://api.lithic.com/v1/auth_rules/d39e680e-af95-47ad-a78e-2415a0c8a81f/apply \
-X POST \
-H "Authorization: YOUR_API_KEY" \
-H "Content-Type: application/json"
-d '
{
"account_tokens": ["ecbd1d58-0299-48b3-84da-6ed7f5bf9ec3"]
}
'
Sample Response
{
"allowed_countries":["USA","CAN"],
"blocked_countries": [],
"allowed_mcc": [],
"blocked_mcc": ["3000","3001"],
"token": "d39e680e-af95-47ad-a78e-2415a0c8a81f",
"program_level": true,
"state": "ACTIVE",
"card_tokens": [],
"account_tokens": ["ecbd1d58-0299-48b3-84da-6ed7f5bf9ec3"]
}
card_tokens (optional) | List of card_token(s) identifying the cards that the Auth Rule will apply to. List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, account_tokens and program_level cannot. However, one of the three parameters must be included. |
account_tokens (optional) | List of account_token(s) identifying the accounts that the Auth Rule will apply to. List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, card_tokens and program_level cannot. However, one of the three parameters must be included. |
program_level (optional) | Boolean indicating whether the Auth Rule should be applied at the program level (true if yes; false if no) String. Permitted values: true , false . Note that if this parameter is included, card_tokens and account_tokens cannot. However, one of the three parameters must be included. |
Remove Auth Rule from Entity
Remove an existing authorization rule from a program, account(s), or card(s). Note that any Auth Rule not applied to an entity will be automatically deactivated (i.e., status will be set to INACTIVE
).
DELETE https://api.lithic.com/v1/auth_rules/remove
Sample Request
curl https://api.lithic.com/v1/auth_rules/remove/ \
-X DELETE \
-H "Authorization: YOUR_API_KEY" \
-H "Content-Type: application/json"
-d '
{
"account_tokens":["ecbd1d58-0299-48b3-84da-6ed7f5bf9ec1"]
}
'
Sample Response
{
"account_tokens": ["ecbd1d58-0299-48b3-84da-6ed7f5bf9ec1"],
"card_tokens": [],
"program_level": false
}
card_tokens (optional) | List of card_token(s) identifying the cards that the Auth Rule will apply to. List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, account_tokens and program_level cannot. However, one of the three parameters must be included. |
account_tokens (optional) | List of account_token(s) identifying the accounts that the Auth Rule will apply to. List. Permitted values: List of 36-digit version 4 UUIDs (including hyphens). Note that if this parameter is included, card_tokens and program_level cannot. However, one of the three parameters must be included. |
program_level (optional) | Boolean indicating whether the Auth Rule should be applied at the program level (true if yes; false if no) String. Permitted values: true , false . Note that if this parameter is included, card_tokens and account_tokens cannot. However, one of the three parameters must be included. |
Auth Rules FAQ
How do Auth Rules at various entity levels interact with each other?
When multiple Auth Rules apply to a given transaction, the most restrictive set of conditions will apply. For example, if an account-level Auth Rule allows transactions in the US and Canada, and a card-level Auth Rule allows for only the US, transactions on that card will be restricted to the US only. In an example with MCC controls, if a program-level Auth Rule blocks MCC 1234 and a card-level Auth Rule allows MCC 5678, then transactions on that card will be permitted only on MCC 5678.What are best practices to manage Auth Rules at scale?
We recommend applying Auth Rules at the highest-level entity possible. For example, if you know that all of your cards will only ever be used on a set of 3 MCCs, we recommend applying that Auth Rule to your program. This will make your Auth Rule easier to manage if you need to add a fourth MCC to the allowed list, as opposed to updating an Auth Rule and applying it to many individual cards.How do country restrictions work for e-commerce?
Country restrictions are based on the country parameter contained in the merchant schema part of a transaction object - i.e., country of card acceptor. This data tends to be highly accurate for card-present transactions, but can be less accurate for e-commerce transactions. For cards that will have a large number of e-commerce use cases, we recommend conducting testing for specific merchants before implementing a country restriction.How do Auth Rules work if I already have ASA implemented?
In the transaction flow, any Auth Rules that apply to your program, account, or card will be checked before you are sent an ASA request. In other words, the transaction will be automatically declined before an ASA request is generated if the transaction's attributes violate any of the configured Auth Rules.How will I know if an authorization on my card program was declined because of an Auth Rule?
In the transaction object, the topline result
field and the result
field contained in the events
array will indicate if it was declined because of an Auth Rule. See this section of our documentation for more detail.
Updated 3 months ago